Projects
Bluetooth Motor Controller
Master
BT-Master Code:
#include “Keypad.h” //Additional Arduino Library
const byte Rows= 4; // Number of rows on the keypad = 4
const byte Cols= 3; // Number of columns on the keypad = 3
// Defining the cols and rows of the keypad
char keymap[Rows][Cols]=
{
{‘#’, ‘0’, ‘*’},
{‘9’, ‘8’, ‘7’},
{‘6’, ‘5’, ‘4’},
{‘3’, ‘2’, ‘1’}
};
byte rPins[Rows]= {A6,A5,A4,A3}; // Rows 0 to 3
byte cPins[Cols]= {A2,A1,A0}; //Columns 0 to 2
// Command for library for keypad
// Initializes an instance of the Keypad class
Keypad kpd= Keypad(makeKeymap(keymap), rPins, cPins, Rows, Cols);
void setup()
{
Serial.begin(9600); // Initializing serial monitor.
Serial1.begin(9600); // Initializing BT communication.
Serial.println(“BTserial started at 9600”);
Serial.println(“”);
}
// If key is pressed, this key is stored in ‘keypressed’ variable
// If key is not equal to ‘NO_KEY’, then this key sent via bluetooth
void loop()
{
char keypressed = kpd.getKey();
if (keypressed != NO_KEY)
{
Serial1.write(keypressed);
}
}
Slave
BT-Slave Code:
// Initializing motor control pins
int pwm = 13;
int dir1 = 30;
int dir2 = 31;
int spd = 0;
void setup() {
Serial.begin(9600); // Initializiang serial monitor for debug
Serial1.begin(9600); // Initializing bluetooth communication
pinMode(pwm, OUTPUT); // Pwm pin = 13
pinMode(dir1, OUTPUT); // Direction logic pin = 30
pinMode(dir2, OUTPUT); // Direction logic pin = 31
// Setting initial direction
digitalWrite(dir1, HIGH);
digitalWrite(dir2, LOW);
}
void loop() {
if(Serial1.available() > 0) {
int input = Serial1.read();
// Received ‘*’ ==> changes direction
if (input == 42) {
digitalWrite(dir1, HIGH);
digitalWrite(dir2, LOW);
Serial.println(“Direction: CCW”);
// Received ‘#’ ==> changes direction
} else if (input == 35) {
digitalWrite(dir1, LOW);
digitalWrite(dir2, HIGH);
Serial.println(“Direction: CW”);
// Received [digit] ==> changes speed dependent on speed
} else {
spd = (input – 48) * 28;//converts user input into speed value
analogWrite(pwm, spd);
Serial.print(“Speed: “);
Serial.println(spd);
}
}
}
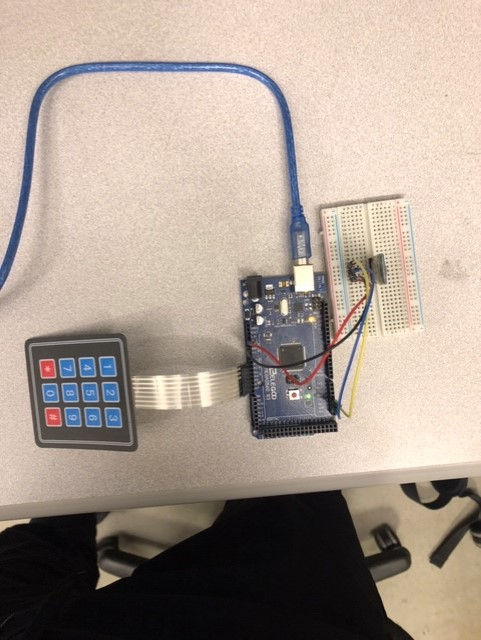
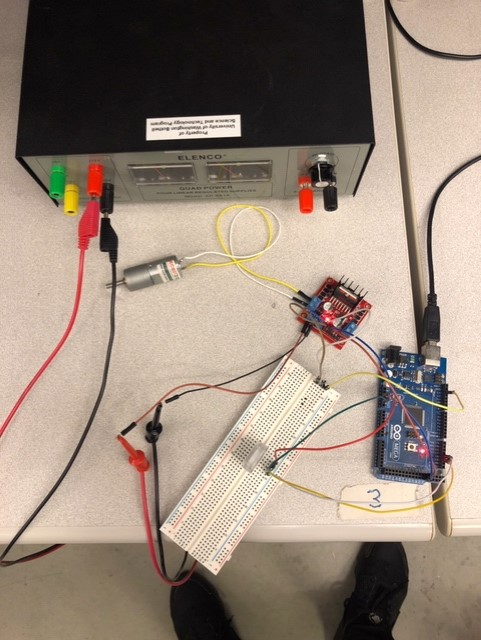